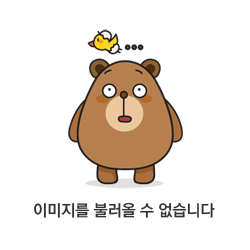
[C#] using 구문
C#에는 using keyword가 있다. C++에도 using 구문이 존재하고 사용법이 다른데 C#은 어떻게 사용하는지 보자.
두 가지 용도로 사용되는데
1. using 지시자
using System.Net;
using System.Net.Http;
using System.Web.Http;
using System.Web.Http.Description;
특정 패키지를 삽입할 때 사용된다. java의 import, C언어의 include와 유사하다.
다음과 같이 별칭으로도 사용이 가능하다.
using System;
using tClass = ConsoleApp1.MyClass.testClass;
namespace ConsoleApp1
{
class Sample
{
static void Main(string[] args)
{
//사용하지 않을 때
ConsoleApp1.MyClass.testClass m1 = new ConsoleApp1.MyClass.testClass();
//사용했을 때
tClass m2 = new tClass();
}
}
}
namespace ConsoleApp1.MyClass
{
class testClass
{
public testClass()
{
Console.WriteLine("NewClass instantiated...");
}
}
}
2. using 명령문
IDisposable 개체의 올바른 사용을 보장하는 편리한 구문을 제공한다.
이것이 무슨 말이냐면, File이나 Font 같은 관리되지 않는 리소스에 액세스 할 때는 사용 후 메모리를 Dispose 해주어야 하는데 이를 using 명령문 안에서 사용하면 자동으로 대행해준다.
일반적으로 using 구문을 사용하지 않는 메모리 해제는 try-finally 구문을 사용하여 다음과 같이 작성하여야 한다.
string manyLines = @"This is line one
This is line two
Here is line three
The penultimate line is line four
This is the final, fifth line.";
{
var reader = new StringReader(manyLines);
try
{
string? item;
do
{
item = reader.ReadLine();
Console.WriteLine(item);
} while (item != null);
}
finally
{
reader?.Dispose();
}
}
using 구문을 사용하면 다음과 같이 코드를 줄일 수 있다.
string manyLines = @"This is line one
This is line two
Here is line three
The penultimate line is line four
This is the final, fifth line.";
using (var reader = new StringReader(manyLines))
{
string? item;
do
{
item = reader.ReadLine();
Console.WriteLine(item);
} while (item != null);
}
// C# 8.0부터는 중괄호가 필요하지 않는다.
using var reader = new StringReader(manyLines);
string? item;
do
{
item = reader.ReadLine();
Console.WriteLine(item);
} while (item != null);
다음 예제와 같이 단일 using 문에서 한 형식의 여러 인스턴스를 선언할 수 있다. 단일 문에서 여러 변수를 선언하는 경우에는 암시적으로 형식화된 변수(var)를 사용할 수 없다.
string numbers = @"One
Two
Three
Four.";
string letters = @"A
B
C
D.";
using (StringReader left = new StringReader(numbers),
right = new StringReader(letters))
{
string? item;
do
{
item = left.ReadLine();
Console.Write(item);
Console.Write(" ");
item = right.ReadLine();
Console.WriteLine(item);
} while (item != null);
}
// C# 8.0에서 다음과 같이 사용 가능.
using StringReader left = new StringReader(numbers),
right = new StringReader(letters);
string? item;
do
{
item = left.ReadLine();
Console.Write(item);
Console.Write(" ");
item = right.ReadLine();
Console.WriteLine(item);
} while (item != null);
<참조>
https://docs.microsoft.com/ko-kr/dotnet/csharp/language-reference/keywords/using-statement
using 문 - C# 참조
using 문(C# 참조) 아티클 07/08/2022 읽는 데 6분 걸림 기여자 14명 이 문서의 내용 --> IDisposable 개체의 올바른 사용을 보장하는 편리한 구문을 제공합니다. C# 8.0부터 using 문은 IAsyncDisposable 개체의 올
docs.microsoft.com
'Back-end' 카테고리의 다른 글
[Redis] Cache 전략 (0) | 2024.07.29 |
---|---|
Redis의 분산락 사용에 대해서 (0) | 2024.07.19 |
[Node.js] NVM으로 여러 버전의 node.js 사용하기 (0) | 2022.07.20 |
[C#] ??(null 병합 연산자 ), ??=(null 병합 할당 연산자), ?.(null 조건 연산자) (0) | 2022.06.20 |
[.NET] .NET Eco System 용어 정리 (ASP.NET, ASP.NET MVC, ADO.NET Core, ADO.NET, cshtml ...) (0) | 2022.06.06 |